YouTube Downloader
— 3 min read
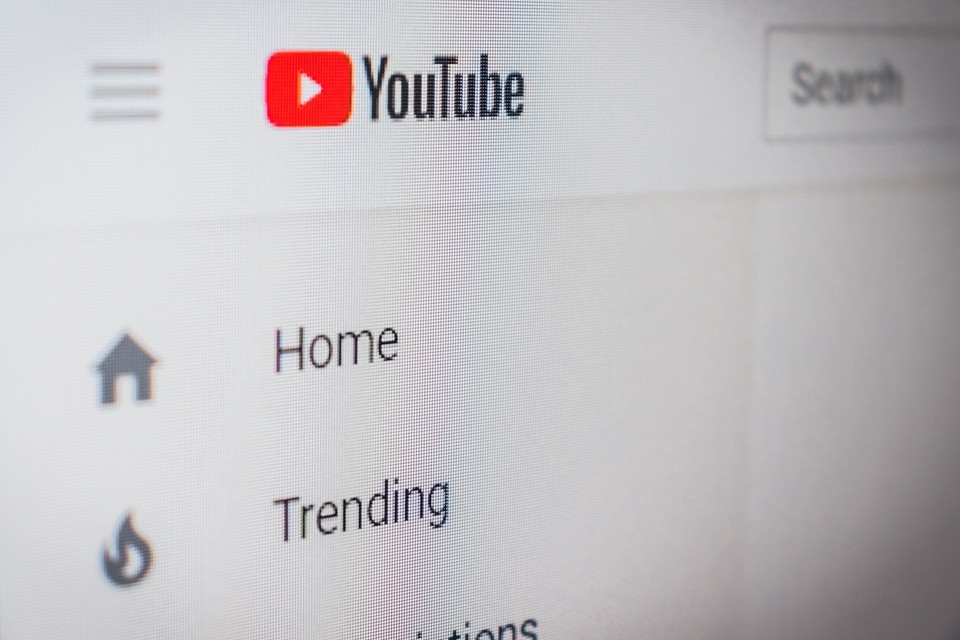
I recently went on a long trip back home to Florida to see family. Flying all the way there can take about 8 hours and I usually watch movies or work on my computer during that time. But before I ended up leaving I decided that I wanted to download some YouTube videos that I had saved.
So I headed over to the videos one by one and clicked on the Download
button where I was hit with this.
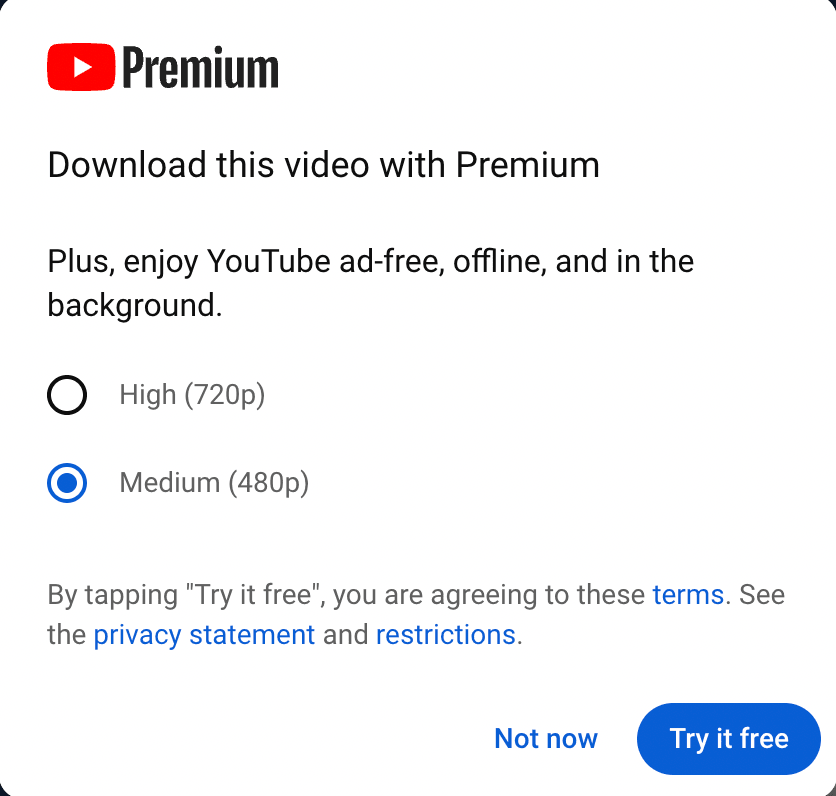
Youtube Premium yikes o.o
I started thinking about how I could get around this and I found websites where you can put a URL and it would give you the mp4 file back. But that wasn't a
great solution since it is so repetitive for each video I wanted to download. Then I started looking for programmatic ways to achieve this and stumbled
upon the pytube
package.
It was pretty simple to get up and running. I created a folder called inputs and made a file named videos.txt where I could dump all of the YouTube URLs I wanted to download. From there I just parsed the list line by line and then download for each link and put the mp4 files into a target directory. Here's what I came up with.
https://www.youtube.com/watch?v=TEkfRSNg7Bk&ab_channel=EpicGardeninghttps://www.youtube.com/watch?v=NvjvzYacgQg&ab_channel=ForgeUtahhttps://www.youtube.com/watch?v=zTQ1kY8xsY8&ab_channel=CloudGuru
from pytube import YouTube
# Video resolution and input/output pathsresolution = "720p"input_file_name = "videos.txt"dest_folder_name = "vods"
# Get the links to downloadwith open(f'./inputs/{input_file_name}', 'r') as inputs: links = inputs.readlines()
# Track if we can't download all of the videoscount = 0total = len(links)
# Download the videosfor link in links: link = link.strip() yt = YouTube(link)
print('Downloading:', yt.title)
# Check for the correct video resolution yd = yt.streams.get_by_resolution(resolution) if not yd: print(f"No {resolution} resolution for the video {yt.title}") continue
yd.download(f"./{dest_folder_name}/") count += 1
print("Complete!")
# I want to know if we don't download all of the videosif count != total: print(f"Downloaded {count} out of {total} videos")
I also added in another python script to remove all of the videos from the target directory when I don't want them anymore.
import os, shutil
dest_folder_name = "vods"
folder = f"./{dest_folder_name}"for filename in os.listdir(folder): file = os.path.join(folder, filename) try: if os.path.isfile(file) or os.path.islink(file): os.unlink(file) elif os.path.isdir(file): shutil.rmtree(file) except Exception as e: print(f"Failed to delete {file}") print(f"Exception: {e}")
The resulting directory looked like this:
If you want to make modification to the resulting resolution you only need to change the variable at the top of the script or you can use the get_highest_resolution()
method
instead of yt.streams.get_by_resolution(resolution)
. I choose to keep it at 720p because I didn't want to download 4k videos and take up all my disk space.
Hope you enjoyed the article and that you got something useful out of it. I found this script really helpful in filling the time on my trip where I didn't have wifi and I will definitely be using it again. Thank you for reading and please share the article if you thought it was helpful!